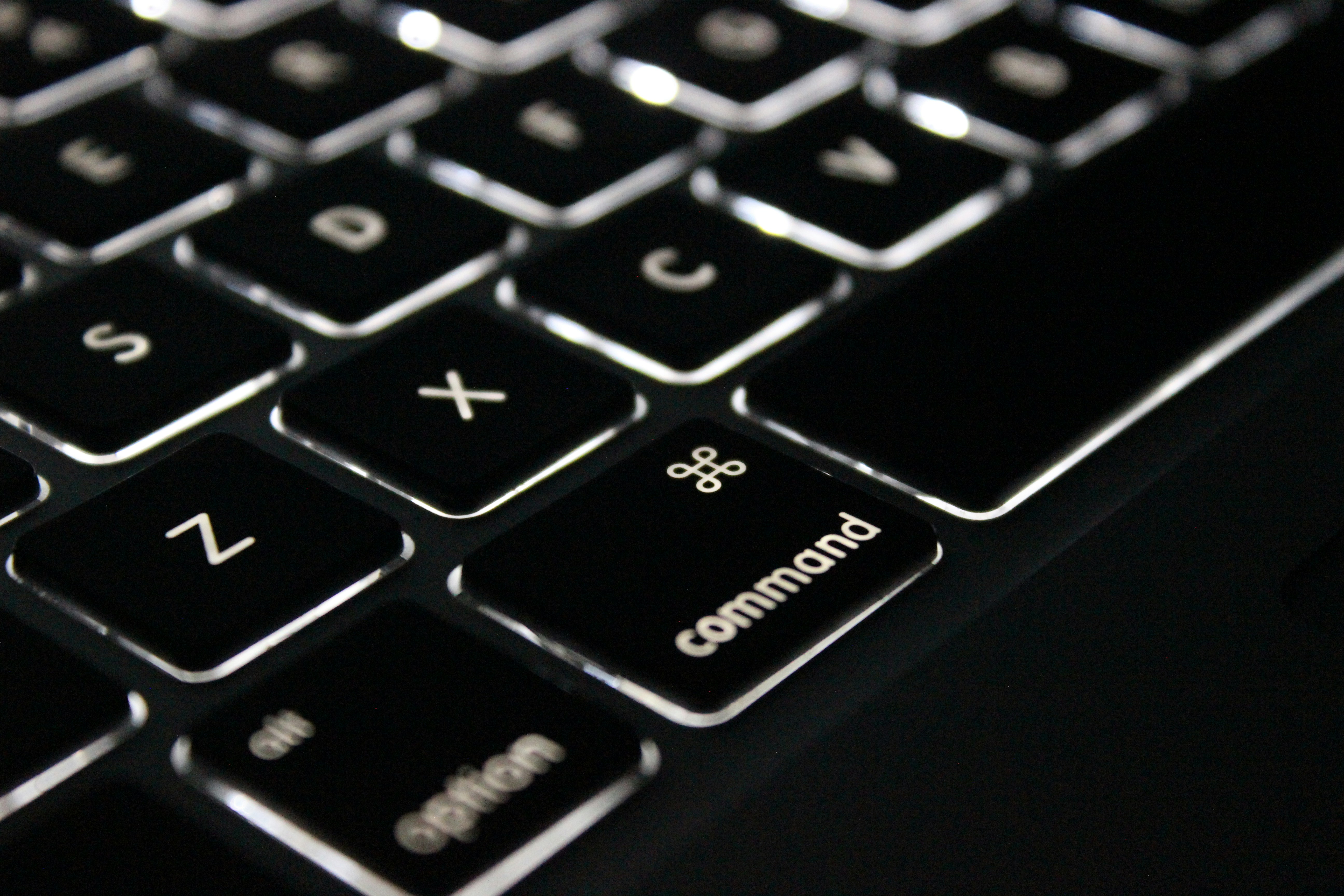
Python Command Pattern: Tutorial for Scalable Projects
By Alyce Osbourne
If you’re tackling complex Python projects and seeking ways to enhance maintainability and flexibility, the Command design pattern could be exactly what you need. This design pattern helps transform a jumble of scattered function calls into well-organized commands that are easy to manage and extend.
In this post, I will explore the fundamentals of the Command design pattern, demonstrate its implementation in Python, and discuss how it can profoundly improve your project’s architecture.
Understanding the Command design pattern
The Command design pattern encapsulates a request as an object, which allows you to parameterize clients with various requests, schedule or queue a request’s execution, and support undoable operations. This pattern is especially valuable in applications that involve menus, queue operations, and transactional behavior.
Advantages of the Command pattern
Imagine you’re developing a web application that allows users to perform actions like submitting forms, fetching data, or processing files. The Command pattern is particularly suited for such scenarios:
- Encapsulation: Commands wrap all the details of an action—including the method call, its arguments, and the owning object—into a standalone object.
- Decoupling: It separates the sender of a request from the receiver that executes it, enhancing both flexibility and component reusability.
- Extensibility: New commands can be introduced without altering existing code, adhering to the open/closed principle.
Key components of the Command pattern
- Command interface: An abstract base that defines the standard interface for all commands.
- Concrete command: This object implements the command interface and encapsulates all details required to perform an action.
- Invoker: This object triggers the command to execute the request. Invokers can either be class or a function.
- Receiver: Knows how to carry out the operations encapsulated by the command.
Implementing the Command pattern in Python
Let’s walk through a simple example to show the Command pattern in Python:
import os
from abc import ABC, abstractmethod
from typing import TextIO
class FileReceiver:
def __init__(self, filename: str):
self.filename = filename
self.file: TextIO | None = None
def open_file(self):
self.file = open(self.filename, 'w')
print(f"File {self.filename} opened.")
def write_file(self, text: str):
if not self.file:
raise Exception("File is not open")
self.file.write(text)
print("Written to file:", text)
def close_file(self):
if self.file:
self.file.close()
print(f"File {self.filename} closed.")
else:
raise Exception("File is not open")
class Command(ABC):
@abstractmethod
def execute(self):
pass
class OpenFileCommand(Command):
def __init__(self, file_receiver: FileReceiver):
self.file_receiver = file_receiver
def execute(self):
self.file_receiver.open_file()
class WriteFileCommand(Command):
def __init__(self, file_receiver: FileReceiver, text: str):
self.file_receiver = file_receiver
self.text = text
def execute(self):
self.file_receiver.write_file(self.text)
class CloseFileCommand(Command):
def __init__(self, file_receiver: FileReceiver):
self.file_receiver = file_receiver
def execute(self):
self.file_receiver.close_file()
class FileOperation:
def __init__(self, commands: list[Command]):
self.commands = commands
def execute_commands(self):
for command in self.commands:
command.execute()
file_receiver = FileReceiver("example.txt")
commands = [
OpenFileCommand(file_receiver),
WriteFileCommand(file_receiver, "Hello, this is a test."),
CloseFileCommand(file_receiver)
]
file_operations = FileOperation(commands)
file_operations.execute_commands()
- FileReceiver manages the file, defining methods for opening, writing to, and closing the file.
- Commands encapsulate each operation on the file, allowing for modular and extendable command creation.
- Invoker (FileOperation) manages and executes the commands and could be enhanced to support operations like undoing, queuing, or logging.
Final thoughts
For Python developers looking to build scalable and maintainable applications, the Command design pattern offers a compelling method to organize and handle requests. By converting function calls into command objects, your code becomes cleaner, easier to extend, and maintain.