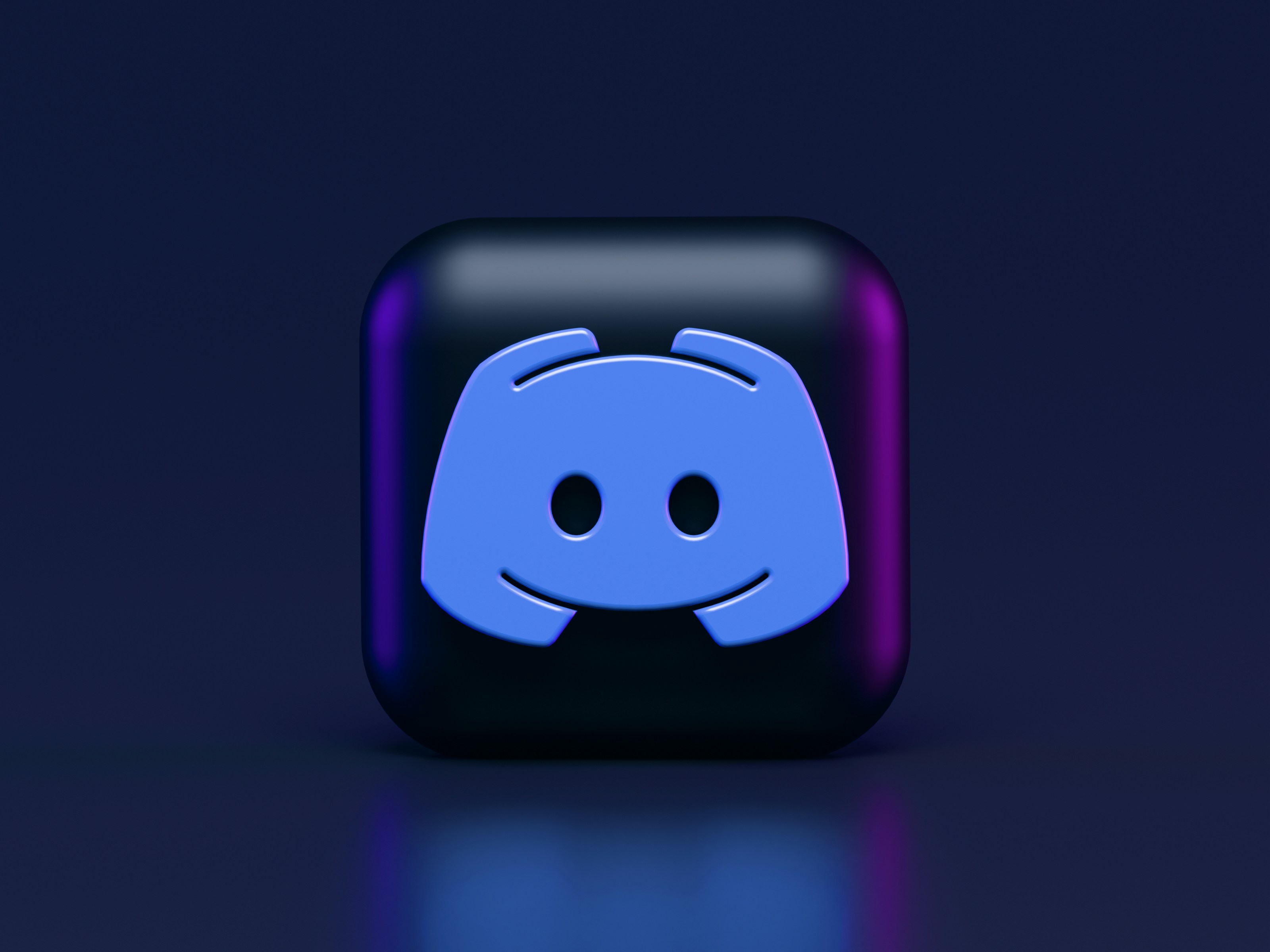
Welcome to the fascinating world of Discord bots! Whether your goal is to improve your server’s capabilities or delve into programming, developing a Discord bot can be a fulfilling pursuit. In this blog post, I will guide you through the basics of building a simple command bot.
Introduction
Discord bots are automated programs designed to perform a multitude of tasks on Discord servers, ranging from moderating conversations to providing entertainment or information. Though the creation process may initially appear complex, with proper guidance, it’s accessible to anyone. This guide focuses on the crucial steps to creating your first Discord bot.
Acquiring your Discord token
The Discord token acts as your bot’s key, enabling it to interact with the Discord API. Follow these steps to obtain your token:
- Navigate to the Discord Developer Portal (https://discord.com/developers/applications) and sign in.
- Select “New Application,” name it, and click “Create.”
- Go to the “Bot” tab and choose “Add Bot.”
- Your bot’s token is visible under the “Token” section. Click “Copy” to save it. Ensure this token remains confidential, as it provides access as if it were your bot.
Implementing a simple prefix command bot
Commands prompt your bot to execute specific actions based on user input. The discord.ext.commands
extension facilitates command creation. Here’s a basic command implementation:
from discord.ext import commands
import discord
import os
bot = commands.Bot(command_prefix='!', intents=discord.Intents.all())
@bot.command()
async def greet(ctx: commands.Context):
await ctx.send('Hello there!')
@bot.event
async def on_ready():
print(f"{discord.utils.oauth_url(bot.user.id, permissions=discord.Permissions.all())}")
bot.run(os.environ["DICORD_BOT_TOKEN"])
- Bot Initialization: The bot is initialized with a command prefix and intents specifying its capabilities.
greet
Command: Activated by typing!greet
, the bot responds with “Hello there!” illustrating a simple interaction.ctx
Parameter: Represents thecommands.Context
, providing details on the event trigger and facilitating responses.
on_ready
Event: Executes when the bot is fully operational, often used to generate an invite link for server addition.bot.run
: The bot is activated with the provided token, which is essential for connecting to Discord. It’s best practice to store your token in an environment variable, rather than using a plain string.
Slash commands
Slash commands offer a streamlined interaction by typing /
followed by the command name, improving the user experience with auto-suggestions and descriptions. These commands leverage Discord’s built-in system and are now recommended for bot interactions.
import discord
from discord.ext import commands
class MyBot(commands.Bot):
async def setup_hook(self):
try:
synced = await self.tree.sync()
except discord.HTTPException as e:
print(f"Failed to sync commands: {e}")
else:
print(f"Synced {len(synced)} commands")
bot = MyBot(command_prefix="!", intents=discord.Intents.all())
@bot.command()
async def greet(ctx: commands.Context, member: discord.Member):
await ctx.send(f'Hello there {member.mention}')
@bot.tree.command(name="ping")
async def ping(ctx: discord.Interaction):
await ctx.response.send_message("Pong!")
@bot.hybrid_command()
async def echo(ctx: commands.Context, message: str):
await ctx.send(message)
@bot.event
async def on_ready():
print(f"{discord.utils.oauth_url(bot.user.id, permissions=discord.Permissions.all())}")
bot.run("YOUR TOKEN HERE")
MyBot
Class: Ensures command synchronization during bot setup, critical for command registration.greet
Prefix Command (!Command): Commands can also accept arguments, and have converters that parse the given text into an object.ping
Slash Command (/Command): Demonstrates a slash command, appearing in Discord’s command palette for easy access.echo
Hybrid Command: Functions as both a text and slash command, echoing user messages and showcasing command flexibility.
Bot hosting
To keep your bot operational, consider these hosting options:
Local hosting
- Pros: Simple and cost-efficient.
- Cons: Limited to your computer’s uptime.
This might be the ideal option for small servers where 100% uptime is not required.
Cloud hosting
- Pros: It ensures 24/7 availability, scalability, and reliability.
- Cons: Potential costs based on usage.
Popular services include Heroku, AWS, and DigitalOcean, each offering varied setup processes for continuous bot operation.
Final thoughts
Discord bots are a great way to provide new features for your community, from code execution to counting, quotes, and games. Discord.py provides many more features than I have shown today, so take time to read through the documentation and go build yourself an awesome Discord bot.