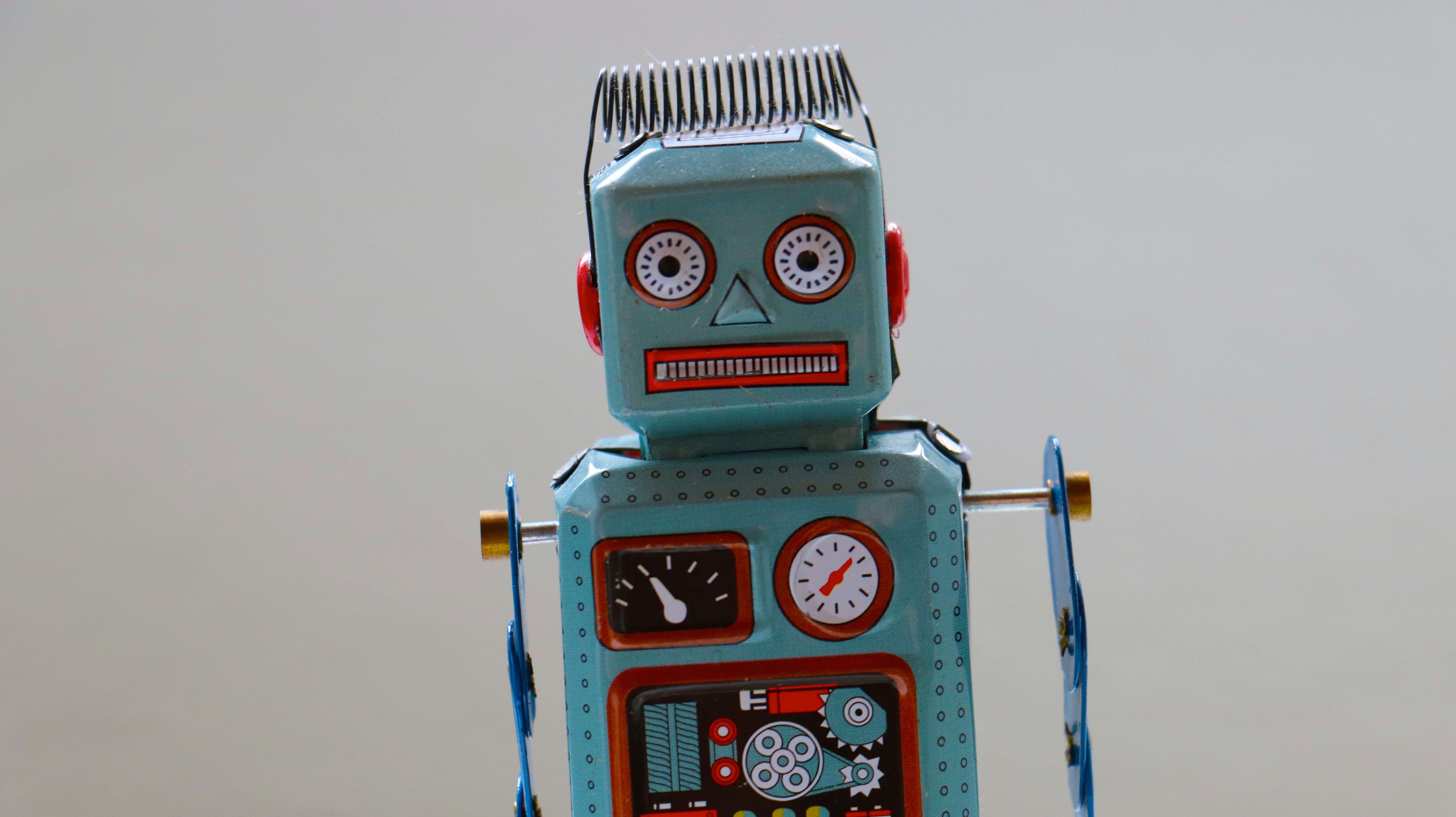
Curious about how to elevate your Python code from merely good to truly exceptional? It’s not about piling up libraries or frameworks; the key is in adopting the sleek principles of functional programming (FP), where code becomes not only cleaner and more efficient but also remarkably easier to maintain. Let’s see why FP is not just another trend but a transformative force for your Python projects.
If you have some experience with Python, you may have come across the concept of functional programming. Rather than being a fleeting trend or technical term, FP signifies a notable change in coding ideology that leads to cleaner and more easily manageable code. This article aims to delve into how integrating FP principles can greatly enhance your Python coding journey.
Decoding functional programming
At its heart, functional programming is about prioritizing the evaluation of functions over altering states and mutable data. It champions expressions over statements, leading to code that’s both readable and straightforward. The predictability of functional code, where outputs depend solely on inputs, eliminates unexpected side effects, making it a popular choice in various coding environments.
Embracing immutability for clearer code
Immutability, a fundamental aspect of FP, involves treating data structures as unchangeable. This approach, while not enforced in Python as in Haskell, can significantly reduce bugs related to state changes. In this model, new data requires creating new objects, which greatly simplifies both debugging and code comprehension.
# Immutable approach
my_tuple = (1, 2, 3)
new_tuple = my_tuple + (4,)
The reliability of pure functions
Pure functions are a staple in FP. These functions always return the same output for the same input and are free from side effects. Designing pure functions in Python enhances their testability and predictability, contributing to more reliable and robust code.
# Pure function
def add_numbers(a, b):
return a + b
result = add_numbers(5, 10)
Higher-order functions: Python’s flexible approach
Python treats functions as first-class citizens. This flexibility allows functions to be assigned to variables, passed as arguments, or returned from other functions. Higher-order functions, which take or return other functions, facilitate elegant patterns like decorators, enhancing functionality in a reusable way.
# Higher-order function
def greet(func):
return func("World")
def hello(name):
return f"Hello, {name}"
print(greet(hello))
Lazy evaluation: list comprehensions and generators
FP advocates for ‘lazy’ evaluation, a concept well-implemented in Python through generators. Generators perform operations only as needed, conserving memory and often enhancing performance. This contrasts with list comprehensions, which eagerly execute operations.
# List comprehension
squares = [x**2 for x in range(10)] # eager
# Generator expression
lazy_squares = (x**2 for x in range(10)) # lazy
The benefits of recursion in functional programming
Recursion, a fundamental concept in functional programming, involves functions invoking themselves. This method provides straightforward solutions to intricate problems and improves the clarity and maintainability of code. Nevertheless, it’s essential to acknowledge Python’s limitation on recursion depth in order to prevent potential errors.
# Recursive function
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
print(factorial(5))
Functional data structures: structuring robust code
FP promotes the use of specific data structures like tuples and immutable lists, which are more predictable and less prone to side effects compared to mutable types. Adopting these structures makes your Python code more functional and robust. Example:
from collections import namedtuple
# NamedTuple
Point = namedtuple('Point', ['x', 'y'])
p = Point(1, 2)
Final thoughts
Implementing functional programming practices in Python consists of intentionally applying principles that enhance the maintainability and resilience of your code. By incorporating concepts like immutability, pure functions, higher-order functions, and functional data structures, you’re not simply following a temporary trend. Instead, you’re honing your Python proficiency and generating code that is durable in the long run. Join a community of dedicated Python enthusiasts who value the sophistication and efficacy of FP.