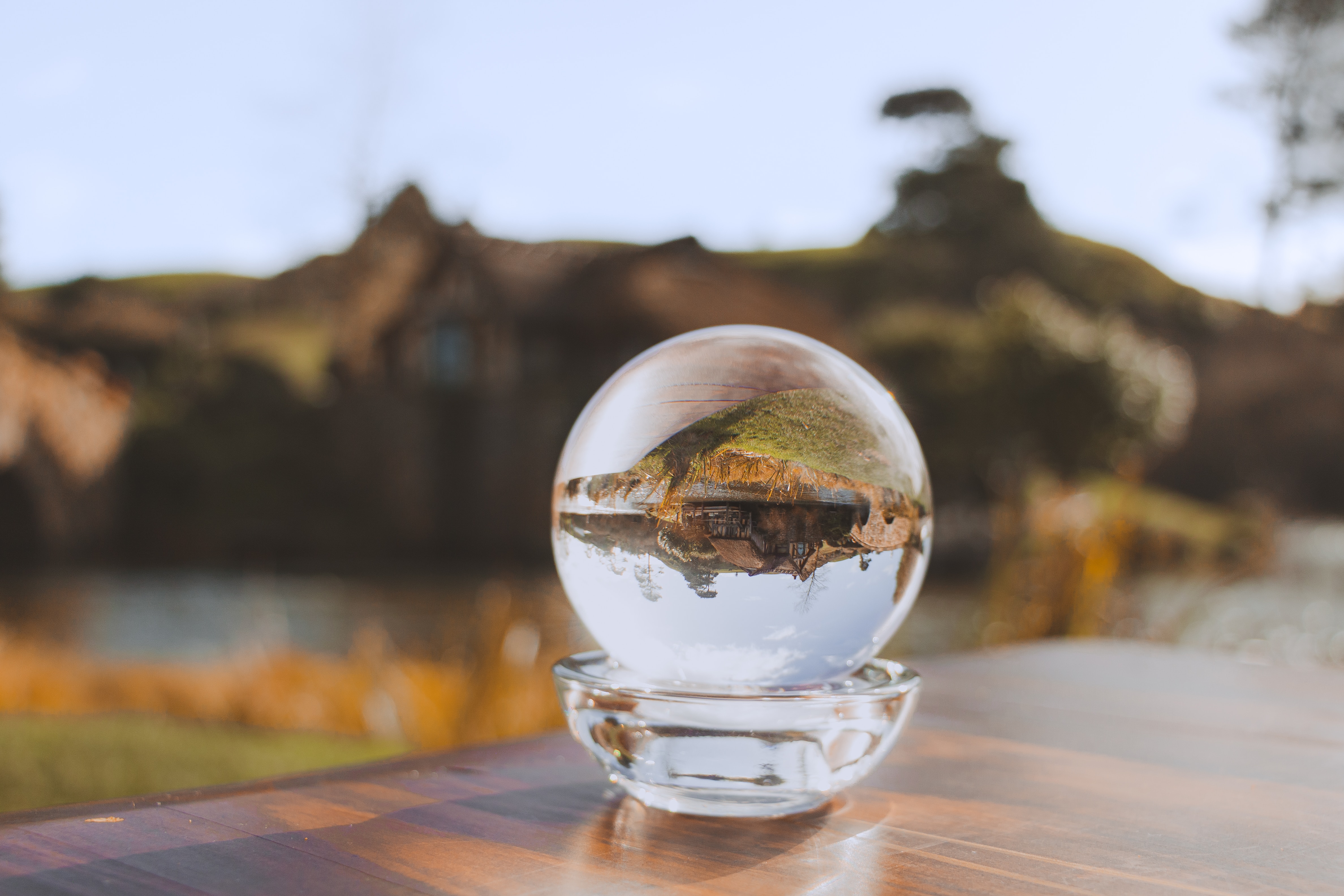
When it comes to software development, dependency inversion is a really cool concept that helps make our code more flexible and easy to work with. Instead of relying on specific implementations, we use abstractions. This little shift in thinking can have a big impact on creating software systems that are easy to modify and extend. In a blog post called “Optimizing Python Code with SOLID Principles” I talked about how we can put dependency inversion into practice. Now, let’s take a deeper look at this idea.
Making things simpler by breaking dependencies with dependency inversion
In normal code, our software becomes tied to specific ways of doing things, and this can make things messy when we need to make changes. But then comes dependency inversion, which breaks these ties to specific implementations. Instead, it encourages us to work with abstractions, making our code more modular and flexible. With this approach, making changes becomes a piece of cake because we can swap out implementations without changing the code that relies on them. The result is code that can adapt easily and be extended, making it a breeze to add new features or components. It’s important to note that dependency inversion works well with dependency injection, which helps keep our code flexible and loosely coupled.
Understanding the dependency inversion principle
In software development, we often find ourselves in situations where we need to add new things to our systems. This can be a pain when our code is tightly tied to specific implementations.
For example, let’s say we have a MessageService
class that sends notifications through email. This class directly relies on the EmailSender
class to send the notifications. This tight coupling makes it hard to add new ways of communication to the system. If we want to add a SmsSender
class to send notifications through SMS, we would need to change the MessageService
class to make it work.
This is not ideal because it goes against the SOLID design principles and can make our code harder to maintain and extend. Let’s look at an example of how Dependency Inversion works in Python:
Without dependency inversion
In the original code, we have a MessageService
class that sends notifications through email using the EmailSender
class. This tightly couples the MessageService
with the specific email implementation, making it difficult to add new ways of communication to the system.
class EmailSender:
def send(self, message: str):
print(f"Sending email: {message}")
class MessageService:
def __init__(self, email_sender: EmailSender):
self.email_sender = email_sender
def send_message(self, message: str):
self.email_sender.send(message)
email_sender = EmailSender()
notification_service = MessageService(email_sender)
notification_service.send_message("Hello World!")
Updated code with dependency inversion
To solve the problem of tight coupling and lack of flexibility, we can refactor the code to use dependency inversion. Instead of relying on concrete implementations like EmailSender
, we introduce an abstract base class called MessageSender
. This abstract class sets the rules for all classes that send messages.
from abc import ABC, abstractmethod
class MessageSender(ABC):
@abstractmethod
def send(self, message: str):
pass
class EmailSender(MessageSender):
def send(self, message: str):
print(f"Sending email: {message}")
class SmsSender(MessageSender):
def send(self, message: str):
print(f"Sending SMS: {message}")
class MessageService:
def __init__(self, message_sender: MessageSender):
self.message_sender = message_sender
def send_message(self, message: str):
self.message_sender.send(message)
email_sender = EmailSender()
notification_service = MessageService(email_sender)
notification_service.send_message("Hello World!")
sms_sender = SmsSender()
notification_service = MessageService(sms_sender)
notification_service.send_message("Hello World!")
See how easy it is now to inject new implementations into our system? This separation of implementation from abstraction is what dependency inversion is all about. It’s important for implementations to follow the rules set by the abstraction.
If you find yourself needing to break those rules, it might be a sign that your design needs some rethinking or that you need to add more functionality to the abstraction. Dependency inversion not only makes your code more adaptable but also encourages good design practices and solid software architecture.
Dependency injection
As I mentioned before, dependency inversion goes hand in hand with dependency injection. They are closely related, and you can’t have dependency inversion without dependency injection. Dependency injection is the process of injecting dependencies into a class or function. Basically, it means that instead of a class managing its own dependencies, they are managed by an external entity. Dependency inversion allows us to specify that the dependencies being injected must follow a certain contract, rather than relying on specific implementations. If you want to learn more about the difference between dependency injection and dependency inversion, check out this video: Dependency INVERSION vs. Dependency INJECTION in Python.
Dependency inversion in Python frameworks
Many Python frameworks and libraries use dependency inversion because they are designed to be easily extended by users. This pattern allows developers to customize and extend various components of the framework, such as ORM models and request handlers. Basically, instead of modifying the framework’s code, developers can create their own implementations and inject them into the framework. This pattern exists in many popular Python frameworks, such as Django, Flask, and SQLAlchemy.
Final thoughts
The concept of dependency inversion has significant implications for the design of software systems in the workplace. It promotes the utilization of abstract frameworks rather than specific implementations, resulting in code that is more versatile, modular, and scalable. Additionally, it encourages the implementation of dependency injection, which further improves the flexibility and minimized interdependence within the codebase. Ultimately, incorporating dependency inversion can facilitate the creation of software systems that are simpler to maintain and expand upon.