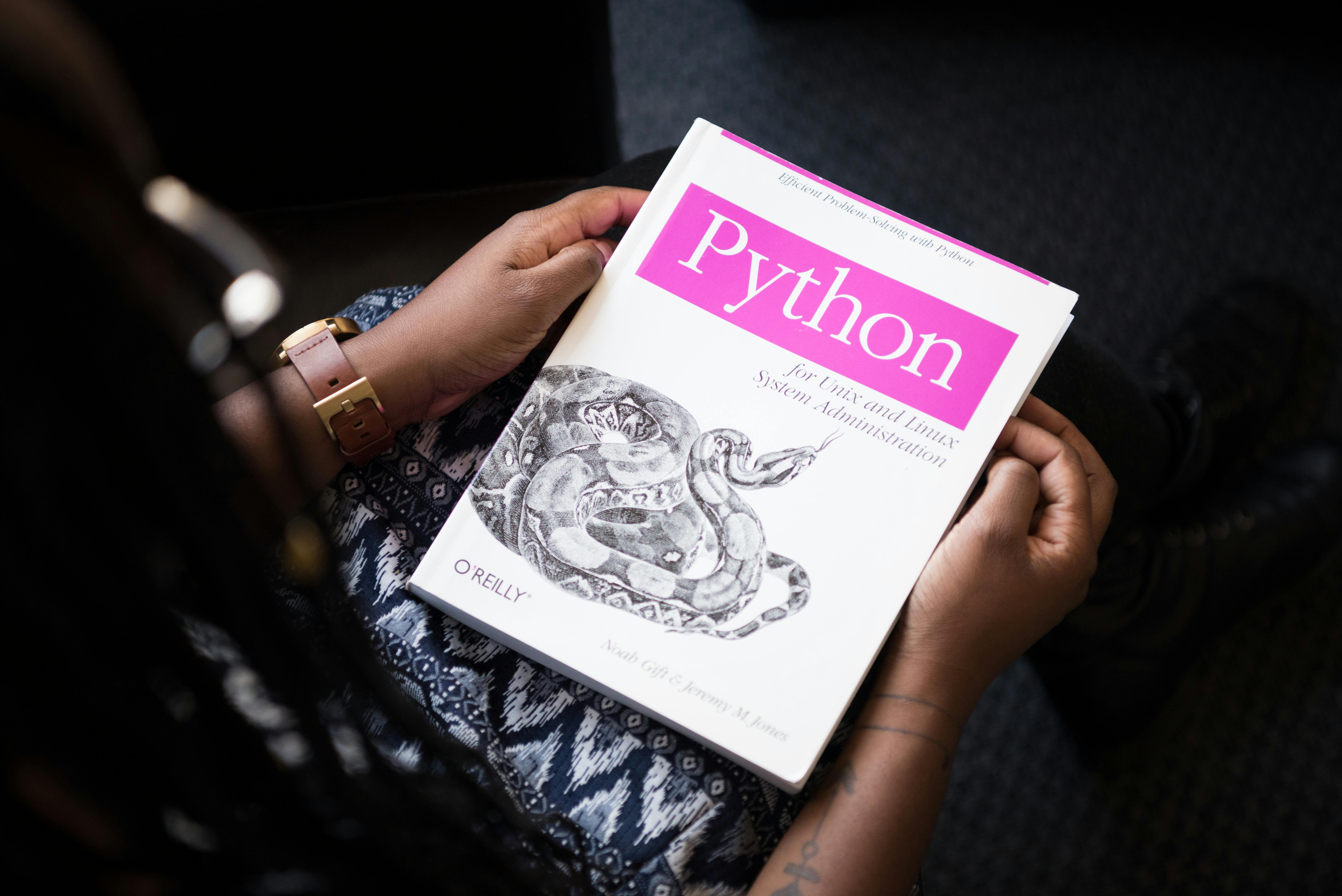
Python, known for its simplicity and readability, encourages developers to write clean, efficient code. However, we all may deviate from the best practices occasionally, especially when faced with tight deadlines and complex project requirements. Here are seven recommendations for improving your Python programming skills.
1. Adhering to PEP 8 standards
The Python Enhancement Proposal (PEP) 8 standards serve as the cornerstone of Python coding conventions. Adherence to these standards ensures that your code is not only readable but also aligns with the collective wisdom of the Python community. It covers essential aspects such as naming conventions and indentation. Tools like flake8
can help enforce these standards across your projects, promoting consistency and readability.
2. Prioritizing error handling
Effective error handling is paramount for developing robust applications. By implementing precise try-except blocks, you can catch and manage exceptions in a controlled manner. Avoid using broad except:
clauses; instead, target specific exceptions to prevent masking underlying issues or silent failures. It’s also advisable to write custom exceptions to add more context to the error messages.
3. Minimizing global variable usage
Excessive reliance on global variables for state management can obfuscate your code’s flow and make it harder to debug. Strive to encapsulate state within classes or pass it through function arguments. When global constants are necessary, ensure they are immutable to minimize side effects. In many cases, this can be solved by making use of concepts such as dependency injection.
4. Opting for simplicity over complexity
Python’s philosophy emphasizes simplicity. Before resorting to complex algorithms or patterns, assess whether a simpler solution exists. This approach not only saves development time but also results in a codebase that is easier to maintain and understand, while also making code easier to debug. Simpler code is also easier to refactor and modularize, and it is frequently easier to make reusable in other parts of your application.
5. Embracing pythonic idioms
Python provides idiomatic constructs for common programming tasks, which can lead to more concise and efficient code. Familiarize yourself with Pythonic idioms, such as using enumerate()
for loops or context managers for resource management, to leverage the full power and elegance of Python.
6. Emphasizing comprehensive testing
Thorough testing is crucial for verifying the functionality of your code and avoiding regressions. Python’s unittest
framework, along with third-party libraries like pytest
, offer robust tools for writing comprehensive tests. Adopting a test-driven development approach can further enhance the design and reliability of your software.
7. Leveraging modern Python features
Python is continuously evolving, with each new version introducing enhancements and features that streamline coding tasks and enhance performance. Keeping your Python skills up-to-date and adopting the latest features can lead to more elegant and efficient code. For example, the assignment expressions (the “walrus operator”) introduced in Python 3.8 allow for cleaner code by enabling variable assignment within expressions.
Python 3.9 brought forward dictionary merge and update operators, simplifying the way dictionaries can be combined and updated. Pattern matching, introduced in Python 3.10, offers a powerful tool for conditional logic that is both readable and efficient. It is beneficial to review the latest Python release notes and experiment with new features to enhance coding practices.
Final thoughts
Embracing these best practices in Python programming goes beyond just making sure your code functions correctly; it’s about writing code that is clean, easy to maintain, and runs efficiently. By following PEP 8 standards, making good use of list comprehensions, focusing on error handling, reducing global variables, opting for simplicity over complexity, utilizing Pythonic idioms, and putting an emphasis on thorough testing, developers can greatly enhance the overall quality of their Python projects. Keep in mind that the objective is to create code that not only meets current needs but also remains sturdy and flexible for future requirements.