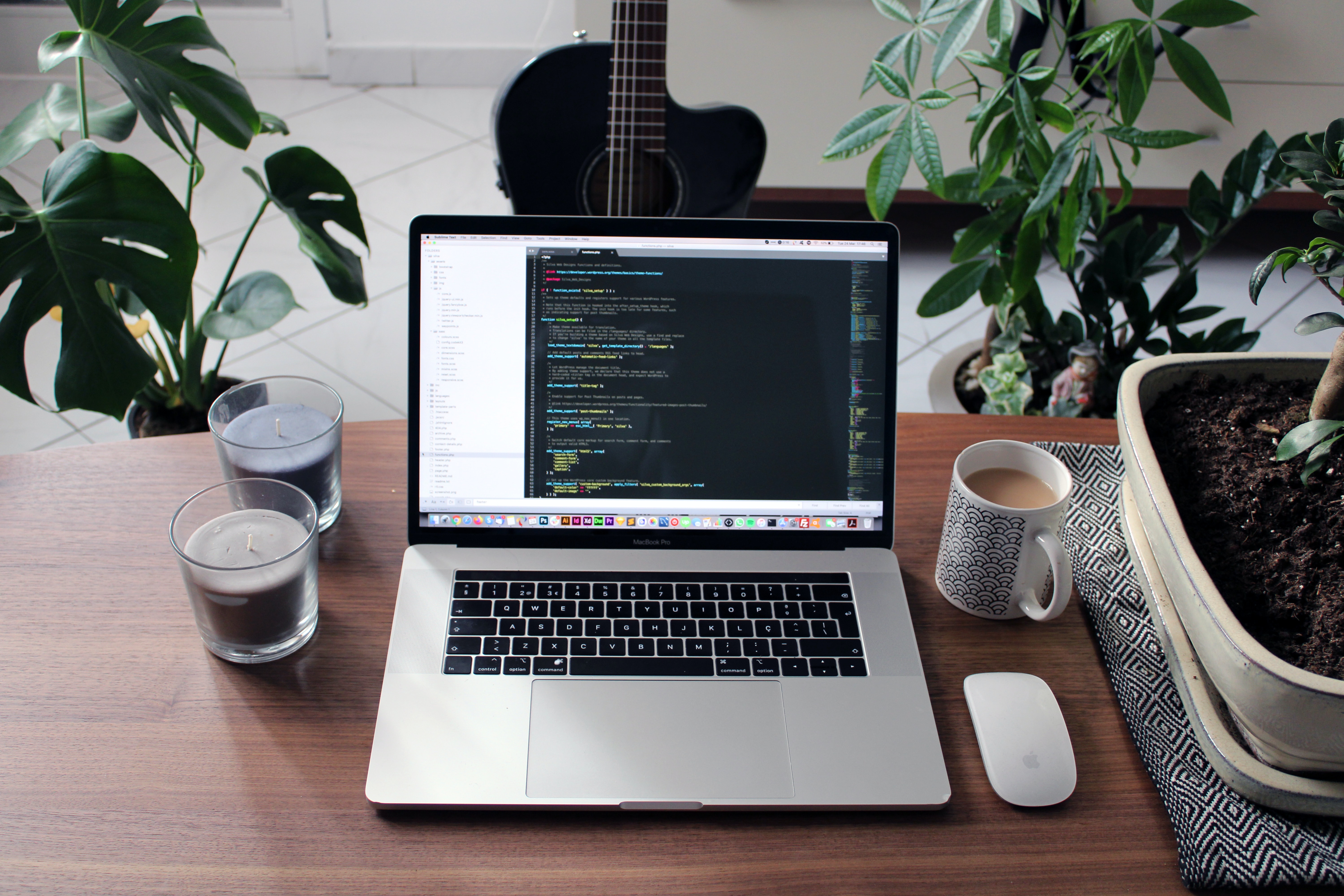
Have you ever wondered how large-scale applications can integrate third-party developments without compromising their core structure? Well, today we’ll be exploring the Plugin Design Pattern, a powerful strategy for decoupling software architectures and facilitating the integration of third-party developments.
What is the plugin design pattern?
The plugin design pattern is an invaluable strategy in software engineering, focusing on the organization of applications to facilitate the integration of ‘plugins’. These plugins are autonomous components that can be added or removed with ease, enabling interaction with the main application to provide distinct features. This design pattern effectively extends the software’s capabilities without necessitating changes to its core structure.
Key principles and advantages of the plugin design pattern:
- Modularity: This pattern divides the software into discrete modules, or components, each responsible for a specific function. This separation simplifies the management, maintenance, and scaling of the software, all while preserving the integrity of other modules.
- Extensibility: Developers can effortlessly augment the application’s capabilities by integrating or detaching plugins, all without modifying the core structure.
- Separation of concerns: Each plugin is dedicated to a particular task or feature, streamlining the process of identifying and addressing issues in an organized manner.
- Scalability: Introducing new plugins or enhancing existing ones is more straightforward than altering a comprehensive structure, facilitating the application’s scalability in response to an expanding user base or increased data and request handling.
- Flexibility: Plugins operate independently, allowing for rapid modification, be it adding, removing, or updating specific functionalities, without significant impact on the main codebase. This autonomy makes the application adaptable to evolving requirements or technologies.
- Maintainability: Isolating functionalities into distinct plugins enables independent development, testing, and maintenance, thereby diminishing the likelihood of bugs and supporting parallel development.
Essential components of the plugin design pattern:
- Host application: This is the primary software or platform that executes the main functions and provides a supportive environment for the plugins. It is usually responsible for the loading and management of plugins, ensuring their operation, and supplying necessary resources or data.
- Plugin interface: Acting as a conduit between the host application and the plugins, the plugin interface delineates the methods, properties, or events that the plugins must implement, thereby guaranteeing consistent interaction with the host.
- Plugins: These are individual modules or components that enhance the functionality of the host application. Compliance with the plugin interface facilitates easy integration and independent development.
Basic structure
from typing import Protocol
class Plugin(Protocol):
def init_app(self, app: "App"):
...
class App(Protocol):
def register_plugin(self, plugin: Plugin):
...
def initialize_plugins(self):
...
This pattern is frequently employed in web applications, with the web application serving as the host and its extensions operating as plugins. The web application provides a mechanism for registering these plugins, while the plugins themselves offer an interface for initializing with the web application.
Example
import flask
import flask_sqlalchemy
app = flask.Flask(__name__)
db = flask_sqlalchemy.SQLAlchemy()
def init_app(app):
db.init_app(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return '<User %r>' % self.username
def main():
init_app(app)
app.run()
if __name__ == '__main__':
main()
In this instance, Flask-SQLAlchemy operates as a plugin for Flask. The plugin provides an init_app
function that initializes the Flask application with the plugin. It also provides an interface for registering models within the database. Notably, the application itself does not require a registry of plugins; instead, the plugins are tasked with registering themselves with the application.
It’s generally advisable to implement a specialized plugin loading system that enables the controlled loading of plugins in a desired sequence. This system can also be used to unload plugins, thereby facilitating the dynamic management of plugins.
Considerations for implementing the plugin design pattern:
- Identifying extensible features: Determine which components of the application are suitable for extension through plugins. Not every feature necessitates a plugin.
- Defining clear interfaces: It’s crucial to establish comprehensive and consistent interfaces for plugins to ensure smooth integration and maintain the host application’s integrity.
- Loose coupling: Maintain a degree of independence between the core application and the plugins to assure stability and resilience, even as the plugins evolve.
- Plugin management: Develop a system for effectively managing plugins, encompassing their loading, unloading, and updating.
- Security: Ensure that plugins are secure and do not compromise the host application’s integrity.
As with the essential components and key principles of plugin design pattern, they all focus on decoupling the code. Making the code maintainable, flexible and just an increase in the life quality of an developer, if you want to read more about how to decoupling your code, check my other blog post.
Where to apply the plugin design pattern
Employ the plugin design pattern when the objective is to extend the functionality of an application without altering its core structure. This pattern is especially advantageous when facilitating third-party developers to enhance the application with additional features.
For more tips on how and where to apply the plugin pattern, check out my video.
Final thoughts
The plugin pattern enhances modularity in software architectures by integrating third-party developments into existing applications without altering the core codebase. It is essential for web applications, as it ensures adaptability and extensibility. Adopting this pattern allows developers to expand an application’s functionality while maintaining a clean core structure. It simplifies the development process and ensures that the application can evolve and adapt to new requirements and technologies over time. The plugin pattern embodies flexibility, scalability, and maintainability, making it indispensable for creating dynamic, extensible, and robust web applications.