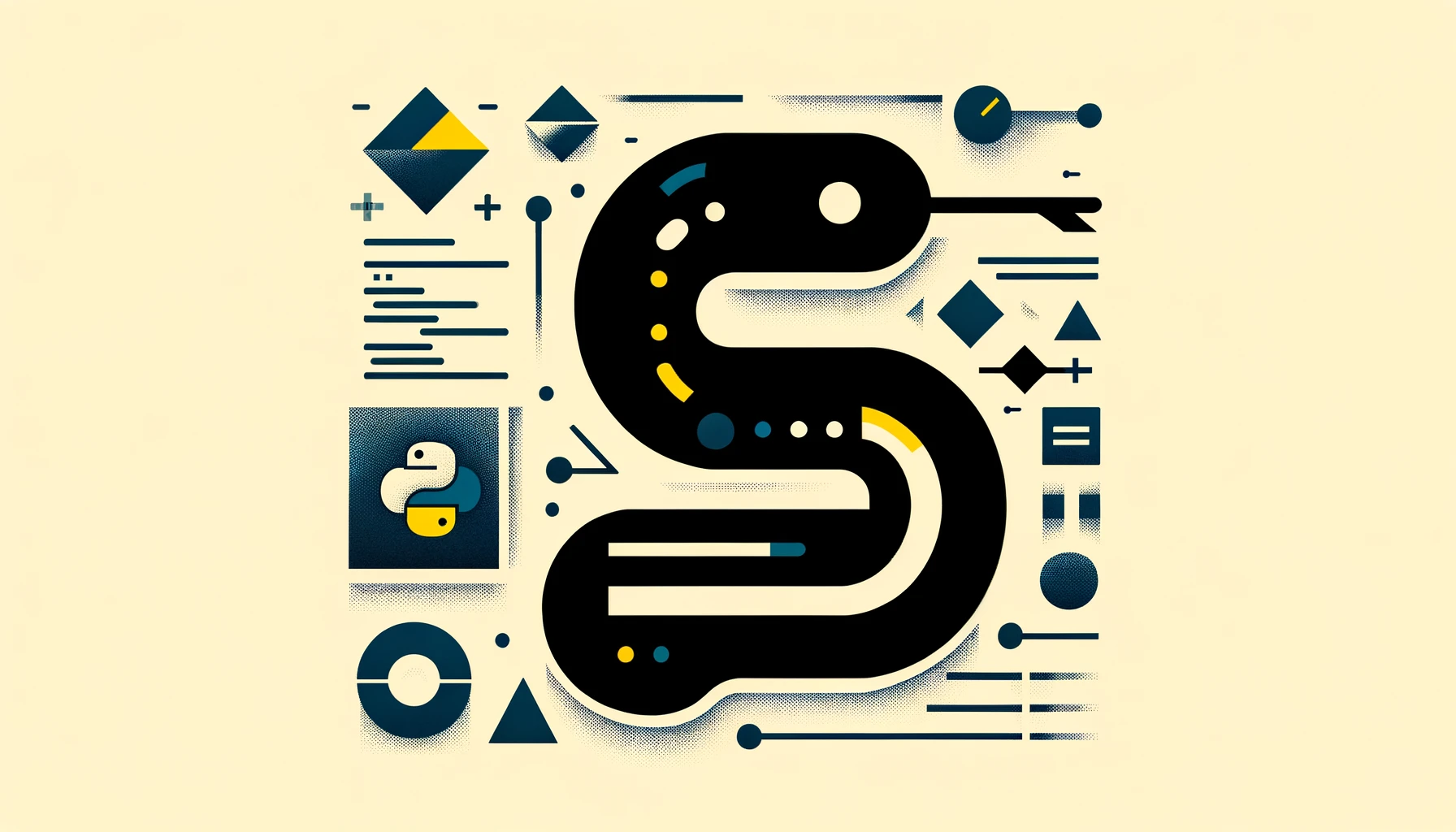
Exception handling is a crucial part of writing solid Python code. It helps developers prepare for and deal with potential errors that might pop up during program execution, ultimately improving the reliability and user experience of the program. Python has made strides over the years in making exception handling more user-friendly and powerful. In this blog post, we’ll take a look at how exception handling works in Python, covering both the traditional methods and newer features.
Getting started with exception handling
When it comes to exception handling in Python, there are four key keywords to keep in mind: try
, except
, else
, and finally
.
try
**:**This is where you place the code that might trigger an exception. Python runs this code and keeps an eye out for any exceptions.except
: If an exception occurs in thetry
block, Python will jump to theexcept
block to handle the error or take the necessary steps for recovery.else
: This block runs if no exceptions were raised in thetry
block. It’s handy for executing code that should only run if thetry
block is successful.finally
: Code within thefinally
block runs no matter what, whether an exception was raised or not. It’s often used for clean-up tasks like closing files or network connections.
try:
# attempt to do something that may raise an exception
except ValueError as e:
# handle exception if raised
else:
# exception wasn't raised, so do something else
finally:
# always do this, regasrdless if an exception was raised or not
Dealing with multiple exceptions
Python lets you catch multiple exceptions in a single except
block using a tuple of exception types or by having multiple except
blocks for different error handling scenarios.
try:
# code that may raise multiple exceptions.
except (TypeError, ValueError) as e:
# handle multiple specific exceptions
except ValueError as e:
# handle any other exceptions
Creating custom exceptions
For more precise control over your exceptions, Python allows you to define custom exceptions. These are typically derived from the built-in Exception
class or one of its subclasses. This is the best way to add extra information to your exceptions and make it more clear what type of problem has occurred.
class MyCustomError(Exception):
"""A custom exception type for specific error handling."""
pass
Use them as you would any other exception: raise MyCustomError
Exception groups
Python 3.11 introduced Exception Groups to handle multiple exceptions raised simultaneously in different parts of a program, especially in concurrent code. This feature makes it easier to group and handle several exceptions together. This is quite useful in cases where you are using concurrent.futures
, as the pools may raise multiple exceptions that all need handling.
try:
# code that may raise multiple exceptions
except* ExceptionGroup as eg:
for exc in eg.exceptions:
# handle individual exceptions in the group
Enhanced traceback information
Python continues to enhance traceback information, making it easier for developers to pinpoint the source of errors. Python 3.10, for example, brought more accurate line numbers in tracebacks and improved error messaging for certain types of exceptions. This includes adding underlines to the exact line of code that failed and suggestions on how to correct them. This new format is pretty reminiscent of how Rust handles its exception messages.
Added context
Python 3.12 has introduced the add_note
 method for adding extra context to exceptions. This can be useful when we want to add extra context when bubbling exceptions up through our application. This is better than raising new exceptions with additional data, as all the original data is preserved.
try:
# Some erronius code
except Exception as e:
e.add_note("More informations")
raise e
Final thoughts
Exception handling is a fundamental and unavoidable aspect of programming, but by making use of the variety of tools Python provides, we can make our exceptions just a little bit exceptional.